Note
This page was generated from circuit-examples//E.Input-output-coupling//42-ResonatorAndLaunchPad.ipynb.
Readout line#
[1]:
#Enables module automatic reload.
#Your notebook will be able to pick up code updates made to qiskit-metal (or other) module code.
%reload_ext autoreload
%autoreload 2
Import key libraries and open the Metal GUI. Also we configure the notebook to enable overwriting of existing components
[2]:
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict, Headings
design = designs.DesignPlanar()
gui = MetalGUI(design)
# If you disable the next line with "overwrite_enabled", then you will need to
# delete a component [<component>.delete()] before recreating it.
design.overwrite_enabled = True
[3]:
from qiskit_metal.qlibrary.terminations.launchpad_wb_coupled import LaunchpadWirebondCoupled
#Explore the options of the LaunchpadWirebondCoupled.
LaunchpadWirebondCoupled.get_template_options(design)
[3]:
{'layer': '1',
'trace_width': 'cpw_width',
'trace_gap': 'cpw_gap',
'coupler_length': '62.5um',
'lead_length': '25um',
'pos_x': '0um',
'pos_y': '0um',
'orientation': '0'}
[4]:
from qiskit_metal.qlibrary.tlines.meandered import RouteMeander
#Explore the options of the RouteMeander.
RouteMeander.get_template_options(design)
[4]:
{'pin_inputs': {'start_pin': {'component': '', 'pin': ''},
'end_pin': {'component': '', 'pin': ''}},
'fillet': '0',
'lead': {'start_straight': '0mm',
'end_straight': '0mm',
'start_jogged_extension': '',
'end_jogged_extension': ''},
'total_length': '7mm',
'chip': 'main',
'layer': '1',
'trace_width': 'cpw_width',
'meander': {'spacing': '200um', 'asymmetry': '0um'},
'snap': 'true',
'prevent_short_edges': 'true'}
[5]:
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
#Explore the options of the OpenToGround.
OpenToGround.get_template_options(design)
[5]:
{'width': '10um',
'gap': '6um',
'termination_gap': '6um',
'pos_x': '0um',
'pos_y': '0um',
'orientation': '0',
'chip': 'main',
'layer': '1'}
[6]:
#Setup the launchpad location and orientation.
launch_options = dict(pos_x='990um', pos_y='2812um', orientation='270', lead_length='30um')
lp = LaunchpadWirebondCoupled(design, 'P4_Q', options = launch_options)
[7]:
#Setup the OpenToGround location and orientation.
otg_options = dict(pos_x='2.5mm', pos_y='0.5mm', orientation='0')
otg = OpenToGround(design, 'open_to_ground', options=otg_options)
[8]:
#After the two QComponents are added to design, connect them with a RouteMeander.
meander_options = Dict(
total_length='10 mm',
fillet='90 um',
lead = dict(start_straight='100um'),
pin_inputs=Dict(
start_pin=Dict(component=lp.name, pin='tie'),
end_pin=Dict(component=otg.name, pin='open')), )
meander = RouteMeander(design, 'bus', options=meander_options)
gui.rebuild()
gui.autoscale()
[9]:
# Get a list of all the qcomponents in QDesign and then zoom on them.
all_component_names = design.components.keys()
gui.zoom_on_components(all_component_names)
[10]:
# Look at the options of the launch pad in QDesign.
lp.options
[10]:
{'layer': '1',
'trace_width': 'cpw_width',
'trace_gap': 'cpw_gap',
'coupler_length': '62.5um',
'lead_length': '30um',
'pos_x': '990um',
'pos_y': '2812um',
'orientation': '270'}
[11]:
# Look at the options of the RouteMeander in QDesign.
meander.options
[11]:
{'pin_inputs': {'start_pin': {'component': 'P4_Q', 'pin': 'tie'},
'end_pin': {'component': 'open_to_ground', 'pin': 'open'}},
'fillet': '90 um',
'lead': {'start_straight': '100um',
'end_straight': '0mm',
'start_jogged_extension': '',
'end_jogged_extension': ''},
'total_length': '10 mm',
'chip': 'main',
'layer': '1',
'trace_width': 'cpw_width',
'meander': {'spacing': '200um', 'asymmetry': '0um'},
'snap': 'true',
'prevent_short_edges': 'true',
'trace_gap': 'cpw_gap',
'_actual_length': '10.0 mm'}
[12]:
#Save screenshot as a .png formatted file.
gui.screenshot()
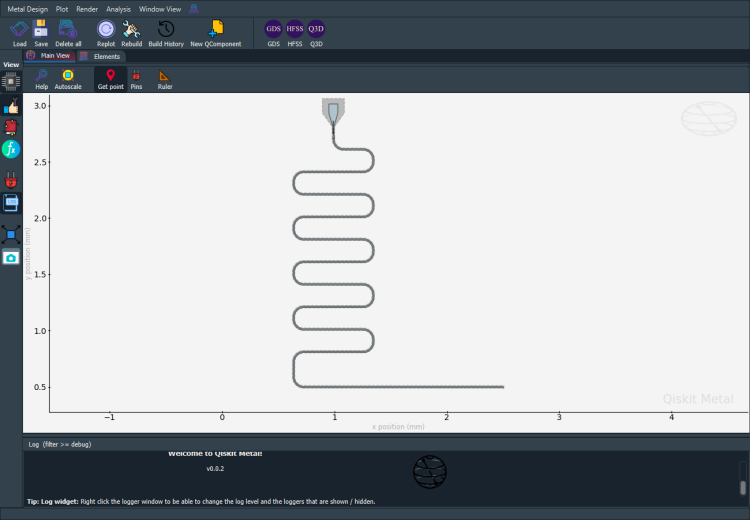
[13]:
# Screenshot the canvas only as a .png formatted file.
gui.figure.savefig('shot.png')
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image('shot.png', **_disp_ops))
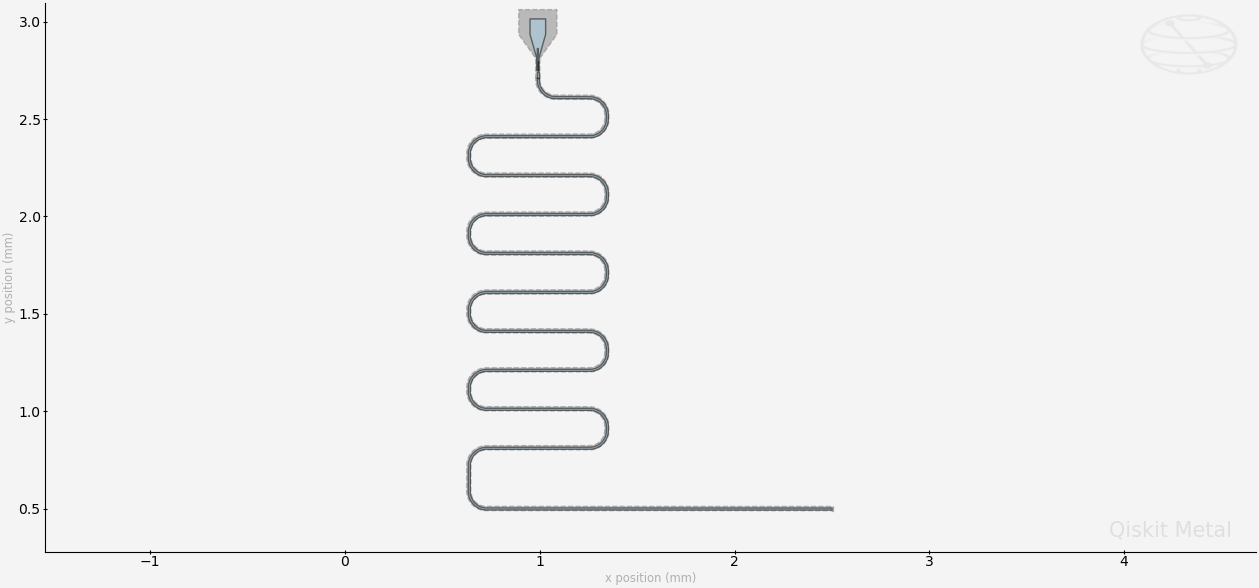
[14]:
# Closing the Qiskit Metal GUI
gui.main_window.close()
[14]:
True
[ ]:
For more information, review the Introduction to Quantum Computing and Quantum Hardware lectures below
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |
|
Lecture Video | Lecture Notes | Lab |