Note
This page was generated from docs/tutorials/10_pvqd.ipynb.
Projected Variational Quantum Dynamics#
The projected Variational Quantum Dynamics (p-VQD) algorithm is a quantum algorithm for real time evolution. It’s a variational algorithm that projects the state at time \(t + \Delta_t\), as calculated with Trotterization, onto a parameterized quantum circuit.
For a quantum state \(|\phi(\theta)\rangle = U(\theta)|0\rangle\) constructed by a parameterized quantum circuit \(U(\theta)\) and a Hamiltonian \(H\), the update rule can be written as
where \(e^{-i\Delta_t H}\) is calculated with a Trotter expansion (using e.g. the PauliEvolutionGate in Qiskit!).
The following tutorial explores the p-VQD algorithm, which is available as the PVQD class. For details on the algorithm, see the original paper: Barison et al. Quantum 5, 512 (2021).
The example we’re looking at is the time evolution of the \(|00\rangle\) state under the Hamiltonian
which is an Ising Hamiltonian on two neighboring spins, up to a time \(T=1\), where we want to keep track of the total magnetization \(M = Z_1 + Z_2\) as an observable.
[1]:
from qiskit.quantum_info import SparsePauliOp
final_time = 1
hamiltonian = SparsePauliOp.from_sparse_list(
[
("ZZ", [0, 1], 0.1),
("X", [0], 1),
("X", [1], 1),
],
num_qubits=2,
)
observable = SparsePauliOp(["ZI", "IZ"])
After defining our Hamiltonian and observable, we need to choose the parameterized ansatz we project the update steps onto. We have different choices here, but for real time evolution an ansatz that contains building blocks of the evolved Hamiltonian usually performs very well.
[2]:
from qiskit.circuit import QuantumCircuit, ParameterVector
theta = ParameterVector("th", 5)
ansatz = QuantumCircuit(2)
ansatz.rx(theta[0], 0)
ansatz.rx(theta[1], 1)
ansatz.rzz(theta[2], 0, 1)
ansatz.rx(theta[3], 0)
ansatz.rx(theta[4], 1)
# you can try different circuits, like:
# from qiskit.circuit.library import EfficientSU2
# ansatz = EfficientSU2(2, reps=1)
ansatz.draw("mpl", style="clifford")
[2]:
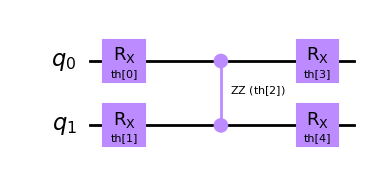
With this ansatz, the \(|00\rangle\) state is prepared if all parameters are 0. Hence we’ll set the initial parameters to \(\theta_0 = 0\):
[3]:
import numpy as np
initial_parameters = np.zeros(ansatz.num_parameters)
Before running the p-VQD algorithm, we need to select the backend and how we want to calculate the expectation values. Here, we’ll perform exact statevector simulations (which are still very fast, as we investigate a 2 qubit system) through the reference primitive implementations found in qiskit.primitives
.
[4]:
from qiskit.primitives import Sampler, Estimator
from qiskit_algorithms.state_fidelities import ComputeUncompute
# the fidelity is used to evaluate the objective: the overlap of the variational form and the trotter step
sampler = Sampler()
fidelity = ComputeUncompute(sampler)
# the estimator is used to evaluate the observables
estimator = Estimator()
Since p-VQD performs a classical optimization in each timestep to determine the best parameters for the projection, we also have to specify the classical optimizer. As a first example we’re using BFGS, which typically works well in statevector simulations, but later we can switch to gradient descent.
[5]:
from qiskit_algorithms.optimizers import L_BFGS_B
bfgs = L_BFGS_B()
Now we can define p-VQD and execute it!
[6]:
from qiskit_algorithms import PVQD
pvqd = PVQD(
fidelity, ansatz, initial_parameters, estimator=estimator, num_timesteps=100, optimizer=bfgs
)
The p-VQD implementation follows Qiskit’s time evolution interface, thus we pack all information of the evolution problem into an input class: the hamiltonian
under which we evolve the state, the final_time
of the evolution and the observables (aux_operators
) we keep track of.
[7]:
from qiskit_algorithms import TimeEvolutionProblem
problem = TimeEvolutionProblem(
hamiltonian, time=final_time, aux_operators=[hamiltonian, observable]
)
And then run the algorithm!
[8]:
result = pvqd.evolve(problem)
print(result)
{ 'aux_ops_evaluated': array([ 0.10531109, -0.82471871]),
'estimated_error': 2.306974043242427e-06,
'evolved_state': <qiskit.circuit.quantumcircuit.QuantumCircuit object at 0x7f568e0bc1f0>,
'fidelities': [ 1.0,
0.9999999975352778,
0.9999999854476362,
0.9999999633016952,
0.9999999998149198,
0.9999999990616129,
0.9999999944518146,
0.9999999993544976,
0.9999999982222058,
0.999999996461848,
0.9999999999401235,
0.9999999999055575,
0.999999999846752,
0.9999999997639674,
0.9999999996573165,
0.9999999995266978,
0.9999999993717236,
0.9999999991916492,
0.9999999989853015,
0.999999998751003,
0.9999999984865068,
0.9999999981889202,
0.9999999978546462,
0.9999999974793188,
0.9999999970577466,
0.9999999965838662,
0.9999999960506925,
0.9999999954502916,
0.999999994773744,
0.9999999940111381,
0.9999999931515537,
0.9999999923775414,
0.9999999913087466,
0.9999999901054976,
0.9999999887529982,
0.999999987533135,
0.999999986189923,
0.9999999847615542,
0.9999999832452297,
0.9999999816071656,
0.9999999799417038,
0.999999978096879,
0.9999999763129154,
0.999999974255315,
0.999999972306668,
0.9999999699029922,
0.9999999675163423,
0.9999999651546538,
0.9999999625738702,
0.9999999600623484,
0.9999999571230342,
0.9999999543144558,
0.9999999515058394,
0.9999999485231986,
0.9999999455935278,
0.9999999424644624,
0.9999999394143853,
0.9999999361759172,
0.999999933024086,
0.9999999297211876,
0.9999999268155843,
0.9999999233786024,
0.999999919774725,
0.9999999168006264,
0.99999991323668,
0.9999999106184974,
0.9999999072916258,
0.9999999038479508,
0.9999999011899022,
0.999999897921698,
0.9999999990098845,
0.9999999989113658,
0.9999999985248682,
0.9999999978110014,
0.9999999967349472,
0.9999999952563786,
0.9999999933479278,
0.9999999909638768,
0.9999999880628654,
0.9999999845895202,
0.9999999804978508,
0.9999999759199913,
0.9999999701581852,
0.9999999641040191,
0.9999999992656146,
0.9999999990293034,
0.999999998556869,
0.9999999978222616,
0.9999999967981168,
0.9999999954557954,
0.9999999939760762,
0.9999999919417746,
0.9999999894850898,
0.9999999865713503,
0.9999999831648652,
0.9999999792290206,
0.9999999752319978,
0.9999999701455526,
0.9999999643932792,
0.9999999999112956,
0.9999999997309256],
'observables': [ array([0.1, 2. ]),
array([0.10010041, 1.99960001]),
array([0.10040158, 1.99840027]),
array([0.10090328, 1.9964014 ]),
array([0.10090144, 1.99360402]),
array([0.10077544, 1.99000929]),
array([0.10052585, 1.98561862]),
array([0.10042343, 1.98042925]),
array([0.1002711 , 1.97444656]),
array([0.10006958, 1.96767296]),
array([0.1000351 , 1.96011965]),
array([0.09999431, 1.95178304]),
array([0.09994794, 1.94266656]),
array([0.09989679, 1.93277394]),
array([0.09984171, 1.92210923]),
array([0.09978361, 1.91067681]),
array([0.09972347, 1.89848136]),
array([0.09966231, 1.88552788]),
array([0.09960122, 1.87182169]),
array([0.09954133, 1.85736838]),
array([0.09948382, 1.8421739 ]),
array([0.09942992, 1.82624445]),
array([0.09938088, 1.80958658]),
array([0.09933803, 1.7922071 ]),
array([0.0993027 , 1.77411313]),
array([0.09927625, 1.75531209]),
array([0.09926011, 1.73581167]),
array([0.09925568, 1.71561986]),
array([0.09926442, 1.69474493]),
array([0.0992878 , 1.67319543]),
array([0.09932728, 1.65098018]),
array([0.09938221, 1.62808815]),
array([0.09945614, 1.6045477 ]),
array([0.09955059, 1.58036851]),
array([0.09966705, 1.55556047]),
array([0.09978497, 1.53010998]),
array([0.09991531, 1.50407332]),
array([0.1000509 , 1.47741369]),
array([0.10019587, 1.45019161]),
array([0.10035011, 1.42236669]),
array([0.10050743, 1.3940039 ]),
array([0.10067835, 1.36505979]),
array([0.10084144, 1.33560017]),
array([0.10102299, 1.30558425]),
array([0.10118735, 1.27506646]),
array([0.1013815 , 1.24404344]),
array([0.10159249, 1.21250065]),
array([0.10180276, 1.18050296]),
array([0.10203349, 1.14801053]),
array([0.10225807, 1.11508468]),
array([0.10251638, 1.08171813]),
array([0.10278825, 1.04788924]),
array([0.1030644 , 1.01367871]),
array([0.10335842, 0.9790334 ]),
array([0.10365293, 0.94403206]),
array([0.10396846, 0.9086248 ]),
array([0.10428453, 0.87288799]),
array([0.10462307, 0.83677401]),
array([0.10496483, 0.80036017]),
array([0.10532909, 0.76359661]),
array([0.1056687 , 0.72654456]),
array([0.10604698, 0.68920742]),
array([0.10646463, 0.65160032]),
array([0.10686561, 0.61371578]),
array([0.10730626, 0.57559126]),
array([0.10770769, 0.53724634]),
array([0.10814843, 0.49869204]),
array([0.10862884, 0.45994397]),
array([0.10908992, 0.42102349]),
array([0.10959035, 0.38194035]),
array([0.10962653, 0.34270135]),
array([0.10964552, 0.30333067]),
array([0.10964633, 0.26384074]),
array([0.10962644, 0.22425309]),
array([0.10958323, 0.18457482]),
array([0.10951391, 0.14483377]),
array([0.10941779, 0.10503142]),
array([0.10929365, 0.06519989]),
array([0.1091406 , 0.02533842]),
array([ 0.10895654, -0.01451983]),
array([ 0.10873973, -0.05437625]),
array([ 0.1085037 , -0.09420056]),
array([ 0.10819701, -0.13398455]),
array([ 0.10787678, -0.17370863]),
array([ 0.10790657, -0.21335407]),
array([ 0.10791108, -0.25290891]),
array([ 0.10788887, -0.29235727]),
array([ 0.10783848, -0.33168332]),
array([ 0.10775845, -0.37087128]),
array([ 0.10764729, -0.40990541]),
array([ 0.10752834, -0.44877267]),
array([ 0.10737451, -0.48745464]),
array([ 0.1071842, -0.5259358]),
array([ 0.10695584, -0.5642007 ]),
array([ 0.10668783, -0.602234 ]),
array([ 0.10637857, -0.64002043]),
array([ 0.10606036, -0.6775498 ]),
array([ 0.1056965, -0.7148017]),
array([ 0.10528536, -0.75176118]),
array([ 0.10530869, -0.78840152]),
array([ 0.10531109, -0.82471871])],
'parameters': [ array([0., 0., 0., 0., 0.]),
array([0.01064827, 0.010873 , 0.0065247 , 0.00935178, 0.00912709]),
array([0.02129654, 0.021746 , 0.01304939, 0.01870356, 0.01825418]),
array([0.03194481, 0.032619 , 0.01957409, 0.02805534, 0.02738127]),
array([0.04144911, 0.04231579, 0.01840757, 0.03855039, 0.03768381]),
array([0.05095342, 0.05201258, 0.01724105, 0.04904544, 0.04798635]),
array([0.06045772, 0.06170936, 0.01607453, 0.0595405 , 0.05828889]),
array([0.0700704 , 0.0715141 , 0.01680252, 0.06994391, 0.06850111]),
array([0.07968308, 0.08131884, 0.0175305 , 0.08034731, 0.07871334]),
array([0.08929576, 0.09112358, 0.01825849, 0.09075072, 0.08892557]),
array([0.09895148, 0.10096712, 0.02005965, 0.10109143, 0.09907813]),
array([0.10860721, 0.11081065, 0.02186081, 0.11143215, 0.1092307 ]),
array([0.11826293, 0.12065418, 0.02366197, 0.12177286, 0.11938327]),
array([0.12791865, 0.13049771, 0.02546314, 0.13211358, 0.12953584]),
array([0.13757437, 0.14034125, 0.0272643 , 0.14245429, 0.13968841]),
array([0.1472301 , 0.15018478, 0.02906546, 0.15279501, 0.14984098]),
array([0.15688582, 0.16002831, 0.03086662, 0.16313572, 0.15999354]),
array([0.16654154, 0.16987185, 0.03266779, 0.17347644, 0.17014611]),
array([0.17619726, 0.17971538, 0.03446895, 0.18381715, 0.18029868]),
array([0.18585299, 0.18955891, 0.03627011, 0.19415787, 0.19045125]),
array([0.19550871, 0.19940245, 0.03807128, 0.20449858, 0.20060382]),
array([0.20516443, 0.20924598, 0.03987244, 0.2148393 , 0.21075639]),
array([0.21482015, 0.21908951, 0.0416736 , 0.22518001, 0.22090895]),
array([0.22447588, 0.22893305, 0.04347476, 0.23552073, 0.23106152]),
array([0.2341316 , 0.23877658, 0.04527593, 0.24586144, 0.24121409]),
array([0.24378732, 0.24862011, 0.04707709, 0.25620216, 0.25136666]),
array([0.25344304, 0.25846365, 0.04887825, 0.26654287, 0.26151923]),
array([0.26309877, 0.26830718, 0.05067941, 0.27688359, 0.2716718 ]),
array([0.27275449, 0.27815071, 0.05248058, 0.2872243 , 0.28182436]),
array([0.28241021, 0.28799424, 0.05428174, 0.29756502, 0.29197693]),
array([0.29206593, 0.29783778, 0.0560829 , 0.30790573, 0.3021295 ]),
array([0.3017307 , 0.30769007, 0.05788166, 0.31825472, 0.31229063]),
array([0.31139547, 0.31754237, 0.05968042, 0.32860371, 0.32245176]),
array([0.32106024, 0.32739467, 0.06147918, 0.33895269, 0.33261289]),
array([0.33072501, 0.33724697, 0.06327794, 0.34930168, 0.34277401]),
array([0.34039973, 0.34710796, 0.06504329, 0.35965891, 0.3529444 ]),
array([0.35006615, 0.35696004, 0.06678943, 0.37000645, 0.36310592]),
array([0.35974285, 0.36682128, 0.0685072 , 0.38036219, 0.37327654]),
array([0.3694112 , 0.37667329, 0.07019961, 0.39070729, 0.38343758]),
array([0.37909023, 0.38653499, 0.07186854, 0.40106075, 0.39360773]),
array([0.3887612 , 0.39638737, 0.07350177, 0.41140229, 0.40376743]),
array([0.39844309, 0.40624979, 0.07511655, 0.42175216, 0.41393612]),
array([0.40811904, 0.41610429, 0.07667996, 0.43208905, 0.42409396]),
array([0.41780532, 0.42596834, 0.07823044, 0.44243329, 0.43425973]),
array([0.42749207, 0.43582991, 0.0797175 , 0.45276534, 0.4444163 ]),
array([0.43717882, 0.44569148, 0.08120457, 0.46309739, 0.45457287]),
array([0.44687645, 0.4555629 , 0.082674 , 0.47343538, 0.46473627]),
array([0.45657115, 0.46542931, 0.08410059, 0.4837594 , 0.47488785]),
array([0.46627686, 0.47530576, 0.08551285, 0.49408917, 0.48504601]),
array([0.47598335, 0.48518039, 0.08687516, 0.50440425, 0.49519211]),
array([0.48568985, 0.49505501, 0.08823748, 0.51471933, 0.5053382 ]),
array([0.49541036, 0.50494237, 0.08957492, 0.52504053, 0.51549157]),
array([0.50512712, 0.51482415, 0.09087394, 0.53534548, 0.52563056]),
array([0.51485788, 0.52471871, 0.09215235, 0.54565603, 0.53577625]),
array([0.5245876 , 0.53461007, 0.09338759, 0.55594907, 0.54590662]),
array([0.53433137, 0.54451427, 0.09460508, 0.56624675, 0.55604274]),
array([0.54407648, 0.55441742, 0.09577928, 0.57652585, 0.56616265]),
array([0.55383616, 0.56433391, 0.09693661, 0.58680855, 0.57628734]),
array([0.5635981 , 0.57425026, 0.09805358, 0.59707159, 0.58639473]),
array([0.57337575, 0.58418101, 0.09915306, 0.6073376 , 0.59650635]),
array([0.58317695, 0.59412988, 0.10018109, 0.61757145, 0.60659119]),
array([0.59297815, 0.60407876, 0.10120912, 0.62780529, 0.61667603]),
array([0.60277936, 0.61402763, 0.10223714, 0.63803914, 0.62676087]),
array([0.61260155, 0.62399444, 0.10320439, 0.6482618 , 0.63683726]),
array([0.62242375, 0.63396125, 0.10417163, 0.65848446, 0.64691366]),
array([0.63227998, 0.64395617, 0.10505362, 0.66866753, 0.6569564 ]),
array([0.64213621, 0.6539511 , 0.10593561, 0.67885059, 0.66699914]),
array([0.65199243, 0.66394602, 0.1068176 , 0.68903365, 0.67704189]),
array([0.66186825, 0.67395718, 0.10763747, 0.69919155, 0.68706269]),
array([0.67174407, 0.68396834, 0.10845735, 0.70934944, 0.69708349]),
array([0.69039436, 0.70179872, 0.10842189, 0.71070025, 0.69925006]),
array([0.7090406 , 0.71963409, 0.10843689, 0.71205274, 0.70141387]),
array([0.72768604, 0.73747139, 0.10850041, 0.71341141, 0.70357369]),
array([0.74632365, 0.75531324, 0.10860925, 0.71477043, 0.70573403]),
array([0.7649628 , 0.77315323, 0.10876054, 0.71614016, 0.70789034]),
array([0.78358959, 0.790998 , 0.10895135, 0.71750742, 0.71005099]),
array([0.80222 , 0.80883764, 0.10918132, 0.71888923, 0.71220762]),
array([0.8208352 , 0.82668151, 0.10944953, 0.72026796, 0.7143712 ]),
array([0.83945343, 0.84451815, 0.10975584, 0.72166358, 0.716532 ]),
array([0.85805435, 0.86235749, 0.11009888, 0.72305751, 0.71870183]),
array([0.87665664, 0.88018745, 0.11047801, 0.7244707 , 0.72087071]),
array([0.89523233, 0.89800858, 0.11090776, 0.725902 , 0.72305479]),
array([0.91380802, 0.91582971, 0.11133751, 0.7273333 , 0.72523886]),
array([0.93236822, 0.93364307, 0.11182698, 0.72878011, 0.72743418]),
array([0.94665133, 0.94858286, 0.11248946, 0.73451723, 0.73251468]),
array([0.96093444, 0.96352266, 0.11315194, 0.74025435, 0.73759518]),
array([0.97521755, 0.97846245, 0.11381443, 0.74599147, 0.74267568]),
array([0.98950066, 0.99340225, 0.11447691, 0.7517286 , 0.74775618]),
array([1.00378377, 1.00834204, 0.11513939, 0.75746572, 0.75283669]),
array([1.01806688, 1.02328184, 0.11580187, 0.76320284, 0.75791719]),
array([1.03234986, 1.03821484, 0.11649208, 0.7689447 , 0.76300653]),
array([1.04663285, 1.05314784, 0.11718229, 0.77468656, 0.76809588]),
array([1.06091583, 1.06808084, 0.1178725 , 0.78042843, 0.77318523]),
array([1.07519881, 1.08301385, 0.1185627 , 0.78617029, 0.77827457]),
array([1.08948179, 1.09794685, 0.11925291, 0.79191215, 0.78336392]),
array([1.10376477, 1.11287985, 0.11994312, 0.79765401, 0.78845326]),
array([1.11803591, 1.12779338, 0.12067322, 0.80341272, 0.7935691 ]),
array([1.13230705, 1.14270691, 0.12140332, 0.80917143, 0.79868494]),
array([1.14657819, 1.15762044, 0.12213343, 0.81493014, 0.80380078]),
array([1.1586098 , 1.16936921, 0.12289866, 0.82293452, 0.81208391]),
array([1.1706414 , 1.18111798, 0.1236639 , 0.83093889, 0.82036704])],
'times': [ 0.0,
0.01,
0.02,
0.03,
0.04,
0.05,
0.06,
0.07,
0.08,
0.09,
0.1,
0.11,
0.12,
0.13,
0.14,
0.15,
0.16,
0.17,
0.18,
0.19,
0.2,
0.21,
0.22,
0.23,
0.24,
0.25,
0.26,
0.27,
0.28,
0.29,
0.3,
0.31,
0.32,
0.33,
0.34,
0.35000000000000003,
0.36,
0.37,
0.38,
0.39,
0.4,
0.41000000000000003,
0.42,
0.43,
0.44,
0.45,
0.46,
0.47000000000000003,
0.48,
0.49,
0.5,
0.51,
0.52,
0.53,
0.54,
0.55,
0.56,
0.5700000000000001,
0.58,
0.59,
0.6,
0.61,
0.62,
0.63,
0.64,
0.65,
0.66,
0.67,
0.68,
0.6900000000000001,
0.7000000000000001,
0.71,
0.72,
0.73,
0.74,
0.75,
0.76,
0.77,
0.78,
0.79,
0.8,
0.81,
0.8200000000000001,
0.8300000000000001,
0.84,
0.85,
0.86,
0.87,
0.88,
0.89,
0.9,
0.91,
0.92,
0.93,
0.9400000000000001,
0.9500000000000001,
0.96,
0.97,
0.98,
0.99,
1.0]}
Now we can have a look at the results, which are stored in a PVQDResult
object. This class has the fields
evolved_state
: The quantum circuit with the parameters at the final evolution time.times
: The timesteps of the time integration. At these times we have the parameter values and evaluated the observables.parameters
: The parameter values at each timestep.observables
: The observable values at each timestep.fidelities
: The fidelity of projecting the Trotter timestep onto the variational form at each timestep.estimated_error
: The estimated error as product of all fidelities.
The energy should be constant in a real time evolution. However, we are projecting the time-evolved state onto a variational form, which might violate this rule. Ideally the energy is still more or less constant. In this evolution here we observe shifts of ~5% of the energy.
[9]:
import matplotlib.pyplot as plt
energies = np.real(result.observables)[:, 0]
plt.plot(result.times, energies, color="royalblue")
plt.xlabel("time $t$")
plt.ylabel("energy $E$")
plt.title("Energy over time")
[9]:
Text(0.5, 1.0, 'Energy over time')
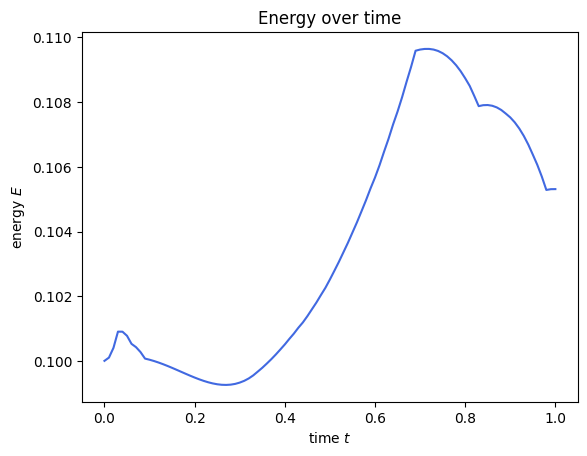
Since we also kept track of the total magnetization of the system, we can plot that quantity too. However let’s first compute exact reference values to verify our algorithm results.
[10]:
import scipy as sc
def exact(final_time, timestep, hamiltonian, initial_state):
"""Get the exact values for energy and the observable."""
O = observable.to_matrix()
H = hamiltonian.to_matrix()
energ, magn = [], [] # list of energies and magnetizations evaluated at timesteps timestep
times = [] # list of timepoints at which energy/obs are evaluated
time = 0
while time <= final_time:
# get exact state at time t
exact_state = initial_state.evolve(sc.linalg.expm(-1j * time * H))
# store observables and time
times.append(time)
energ.append(exact_state.expectation_value(H).real)
magn.append(exact_state.expectation_value(observable).real)
# next timestep
time += timestep
return times, energ, magn
[11]:
from qiskit.quantum_info import Statevector
initial_state = Statevector(ansatz.assign_parameters(initial_parameters))
exact_times, exact_energies, exact_magnetizations = exact(
final_time, 0.01, hamiltonian, initial_state
)
[12]:
magnetizations = np.real(result.observables)[:, 1]
plt.plot(result.times, magnetizations.real, color="crimson", label="PVQD")
plt.plot(exact_times, exact_magnetizations, ":", color="k", label="Exact")
plt.xlabel("time $t$")
plt.ylabel(r"magnetization $\langle Z_1 Z_2 \rangle$")
plt.title("Magnetization over time")
plt.legend(loc="best")
[12]:
<matplotlib.legend.Legend at 0x7f568df45e50>
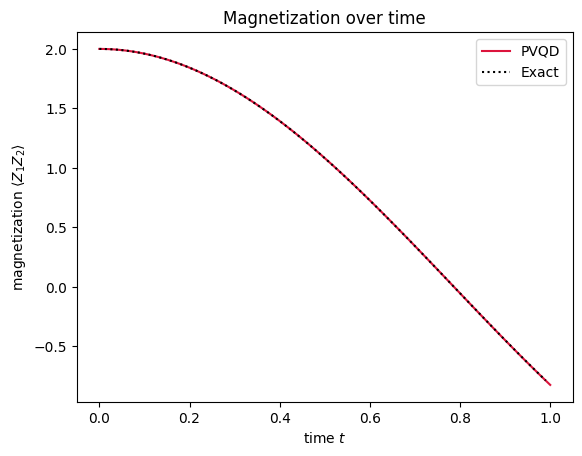
Looks pretty good!
Gradient-based optimizations#
The PVQD
class also implements parameter-shift gradients for the loss function and we can use a gradient descent optimization routine
Here we’re using a learning rate of
and 80 optimization steps in each timestep.
[13]:
from qiskit_algorithms.optimizers import GradientDescent
maxiter = 80
learning_rate = 0.1 * np.arange(1, maxiter + 1) ** (-0.602)
gd = GradientDescent(maxiter, lambda: iter(learning_rate))
[14]:
pvqd.optimizer = gd
The following cell would take a few minutes to run for 100 timesteps, so we reduce them here.
[15]:
n = 10
pvqd.num_timesteps = n
problem.time = 0.1
[16]:
result_gd = pvqd.evolve(problem)
[17]:
energies_gd = np.real(result_gd.observables)[:, 0]
plt.plot(result.times[: n + 1], energies[: n + 1], "-", color="royalblue", label="BFGS")
plt.plot(result_gd.times, energies_gd, "--", color="royalblue", label="Gradient descent")
plt.plot(exact_times[: n + 1], exact_energies[: n + 1], ":", color="k", label="Exact")
plt.legend(loc="best")
plt.xlabel("time $t$")
plt.ylabel("energy $E$")
plt.title("Energy over time")
[17]:
Text(0.5, 1.0, 'Energy over time')
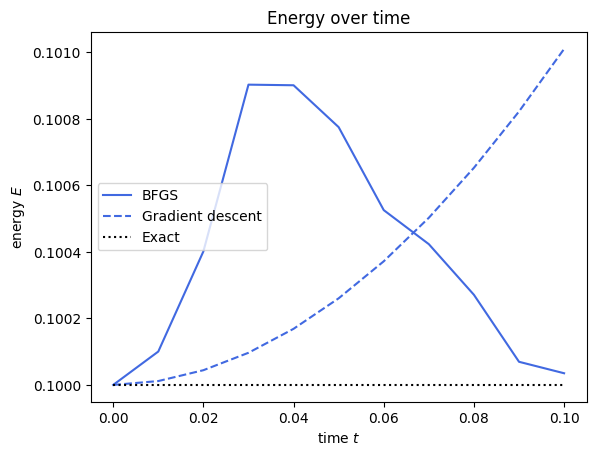
We can observe here, that the energy does vary quite a bit! But as we mentioned before, p-VQD does not preserve the energy.
[18]:
magnetizations_gd = np.real(result_gd.observables)[:, 1]
plt.plot(result.times[: n + 1], magnetizations[: n + 1], "-", color="crimson", label="BFGS")
plt.plot(result_gd.times, magnetizations_gd, "--", color="crimson", label="Gradient descent")
plt.plot(exact_times[: n + 1], exact_magnetizations[: n + 1], ":", color="k", label="Exact")
plt.legend(loc="best")
plt.xlabel("time $t$")
plt.ylabel(r"magnetization $\langle Z_1 + Z_2 \rangle$")
plt.title("Magnetization over time")
[18]:
Text(0.5, 1.0, 'Magnetization over time')
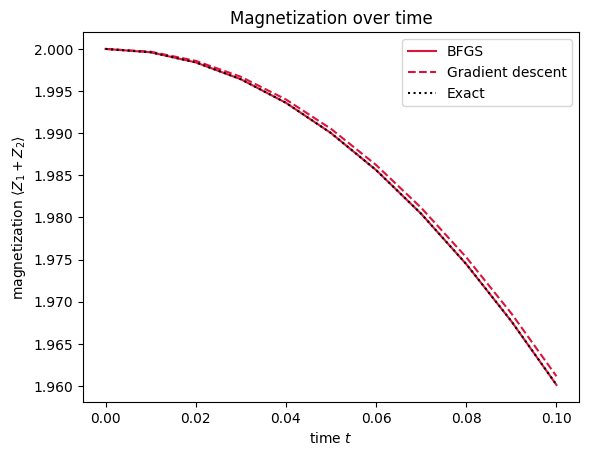
The magnetization, however, is computed very precisely.
[19]:
import tutorial_magics
%qiskit_version_table
%qiskit_copyright
Version Information
Software | Version |
---|---|
qiskit | 1.0.2 |
qiskit_algorithms | 0.3.0 |
qiskit_aer | 0.14.0.1 |
System information | |
Python version | 3.8.18 |
OS | Linux |
Wed Apr 10 17:19:41 2024 UTC |
This code is a part of a Qiskit project
© Copyright IBM 2017, 2024.
This code is licensed under the Apache License, Version 2.0. You may
obtain a copy of this license in the LICENSE.txt file in the root directory
of this source tree or at http://www.apache.org/licenses/LICENSE-2.0.
Any modifications or derivative works of this code must retain this
copyright notice, and modified files need to carry a notice indicating
that they have been altered from the originals.